Managing Continuous Glucose Monitor (CGM) data can be a complex task, but with Python, it becomes a more accessible and efficient process. This guide will walk you through the steps to effectively process and analyze CGM data, offering a range of tips and tricks to enhance your data management skills.
Getting Started with CGM Data Processing

Before diving into the specifics, let's understand the basics of CGM data and its importance in healthcare.
What is CGM Data?

CGM data refers to the continuous stream of glucose level readings obtained from a small sensor inserted under the skin. These sensors provide real-time glucose measurements, offering valuable insights into an individual's glucose trends and patterns.
Why Process CGM Data with Python?

Python is a versatile and powerful programming language known for its simplicity and extensive libraries. It provides an ideal platform for data analysis, visualization, and automation, making it an excellent choice for CGM data processing.
Step-by-Step Guide to CGM Data Processing
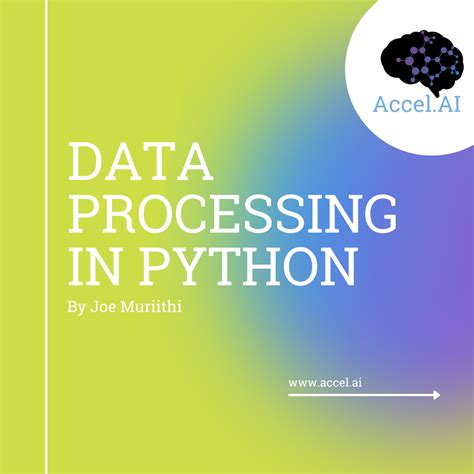
Step 1: Collecting and Importing CGM Data

The first step is to collect CGM data from your device or application. Most CGM manufacturers provide data export options, allowing you to download the data in various formats, such as CSV or JSON.
Once you have the data, use Python's built-in libraries like pandas
to import and organize the data into a structured format.
import pandas as pd
# Load CGM data from CSV file
cgm_data = pd.read_csv('cgm_data.csv')
Step 2: Data Cleaning and Preprocessing

Raw CGM data often contains missing values, outliers, and inconsistencies. It's crucial to clean and preprocess the data to ensure accurate analysis.
Handling Missing Values
Identify and handle missing values using techniques like imputation or dropping rows with missing data.
# Impute missing values with the mean of the 'glucose' column
cgm_data['glucose'].fillna(cgm_data['glucose'].mean(), inplace=True)
Outlier Detection and Removal
Detect and remove outliers that may skew your analysis. You can use statistical methods or visualization techniques to identify outliers.
# Calculate the 1st and 3rd quartiles
Q1 = cgm_data['glucose'].quantile(0.25)
Q3 = cgm_data['glucose'].quantile(0.75)
# Calculate the interquartile range (IQR)
IQR = Q3 - Q1
# Define outliers as values outside 1.5*IQR
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
# Remove outliers
cgm_data = cgm_data[(cgm_data['glucose'] >= lower_bound) & (cgm_data['glucose'] <= upper_bound)]
Data Normalization
Normalize your data to ensure consistency and comparability across different CGM devices or individuals.
# Normalize glucose values to a range of 0 to 1
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
cgm_data['normalized_glucose'] = scaler.fit_transform(cgm_data[['glucose']])
Step 3: Data Exploration and Visualization

Visualizing your CGM data is essential for understanding trends, patterns, and anomalies. Python offers a wide range of visualization libraries, such as matplotlib
and seaborn
.
Time Series Plots
Create time series plots to visualize glucose levels over time. This helps identify daily, weekly, or monthly patterns.
import matplotlib.pyplot as plt
# Create a time series plot
plt.plot(cgm_data['timestamp'], cgm_data['glucose'], label='Glucose Levels')
plt.xlabel('Timestamp')
plt.ylabel('Glucose Level (mg/dL)')
plt.title('Glucose Levels Over Time')
plt.legend()
plt.show()
Histogram and Density Plots
Use histograms and density plots to analyze the distribution of glucose levels.
import seaborn as sns
# Create a histogram
sns.histplot(cgm_data['glucose'], kde=True)
plt.xlabel('Glucose Level (mg/dL)')
plt.ylabel('Frequency')
plt.title('Distribution of Glucose Levels')
plt.show()
Interactive Visualizations
For more interactive visualizations, consider using libraries like plotly
or bokeh
.
import plotly.express as px
# Create an interactive line plot
fig = px.line(cgm_data, x='timestamp', y='glucose', title='Glucose Levels Over Time')
fig.show()
Step 4: Data Analysis and Insights

With your data cleaned and visualized, it's time to extract meaningful insights and perform advanced analysis.
Calculating Statistical Measures
Compute various statistical measures, such as mean, median, standard deviation, and percentiles, to understand the central tendency and variability of glucose levels.
# Calculate statistical measures
mean_glucose = cgm_data['glucose'].mean()
median_glucose = cgm_data['glucose'].median()
std_dev_glucose = cgm_data['glucose'].std()
Identifying Trends and Patterns
Analyze trends and patterns in your CGM data to identify potential issues or areas of improvement.
# Calculate the rolling mean to identify trends
rolling_mean = cgm_data['glucose'].rolling(window=24, min_periods=1).mean()
# Plot the rolling mean
plt.plot(cgm_data['timestamp'], rolling_mean, label='Rolling Mean')
plt.xlabel('Timestamp')
plt.ylabel('Glucose Level (mg/dL)')
plt.title('Rolling Mean of Glucose Levels')
plt.legend()
plt.show()
Comparing Different Time Periods
Compare glucose levels across different time periods, such as before and after a specific event or intervention.
# Split the data into two periods
period1 = cgm_data[cgm_data['timestamp'] < '2023-06-01']
period2 = cgm_data[cgm_data['timestamp'] >= '2023-06-01']
# Calculate the mean glucose for each period
mean_period1 = period1['glucose'].mean()
mean_period2 = period2['glucose'].mean()
# Compare the means
print(f"Mean Glucose in Period 1: {mean_period1:.2f} mg/dL")
print(f"Mean Glucose in Period 2: {mean_period2:.2f} mg/dL")
Step 5: Advanced Analysis and Modeling

For more advanced analysis, you can explore machine learning techniques and predictive modeling.
Time Series Forecasting
Predict future glucose levels using time series forecasting models like ARIMA or Prophet.
from statsmodels.tsa.arima.model import ARIMA
# Fit an ARIMA model
model = ARIMA(cgm_data['glucose'], order=(2, 1, 1))
model_fit = model.fit()
# Forecast future glucose levels
forecast = model_fit.forecast(steps=30)
print(forecast)
Machine Learning for Glucose Prediction
Train machine learning models to predict glucose levels based on various features.
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestRegressor
# Split data into training and testing sets
X = cgm_data.drop('glucose', axis=1)
y = cgm_data['glucose']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest Regressor
model = RandomForestRegressor(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Evaluate the model
score = model.score(X_test, y_test)
print(f"Model Accuracy: {score:.2f}")
Tips and Tricks for Efficient CGM Data Processing

Tip 1: Data Quality Assurance

Implement rigorous data quality checks to ensure the accuracy and reliability of your CGM data. Validate data against known ranges and identify any potential issues.
Tip 2: Automated Data Processing
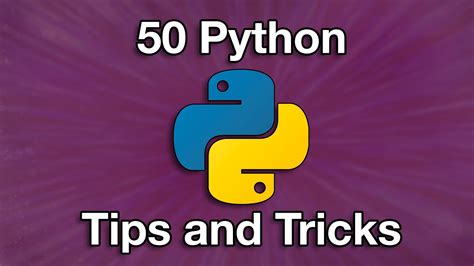
Automate your data processing pipeline to save time and effort. Use Python's scheduling libraries to run your data processing scripts at regular intervals.
Tip 3: Data Integration

Combine CGM data with other relevant data sources, such as diet, exercise, or medication information, to gain a more comprehensive understanding of an individual's health.
Tip 4: Feature Engineering
Create new features from your CGM data to improve the performance of your models. For example, calculate the rate of change in glucose levels or identify periods of high variability.
Tip 5: Model Evaluation and Validation
Evaluate and validate your models thoroughly. Use cross-validation techniques and performance metrics to assess the effectiveness of your predictive models.
Tip 6: Visualize Model Performance
Visualize the performance of your models to better understand their strengths and weaknesses. Create confusion matrices, ROC curves, or lift charts to evaluate classification models.
Tip 7: Continuous Learning and Improvement
Stay updated with the latest advancements in CGM data processing and machine learning. Attend conferences, join online communities, and explore new libraries and techniques to enhance your skills.
Conclusion

Processing CGM data with Python offers a powerful and flexible approach to managing and analyzing glucose levels. By following this guide, you can efficiently collect, clean, visualize, and analyze CGM data, gaining valuable insights into an individual's glucose trends and patterns. Remember to adapt and customize your data processing pipeline based on your specific needs and the nature of your CGM data.
Frequently Asked Questions

How often should I collect CGM data for analysis?
+The frequency of data collection depends on your specific use case and the nature of the CGM device. In general, collecting data at regular intervals, such as hourly or daily, is recommended to capture accurate trends.
What are some common challenges in CGM data processing?
+Common challenges include handling missing data, dealing with outliers, and ensuring data consistency across different devices or individuals. It’s important to implement robust data cleaning and preprocessing techniques.
How can I visualize glucose trends over time?
+Create time series plots using libraries like matplotlib
or seaborn
. Plot the glucose levels against timestamps to visualize trends and patterns.
Can I use machine learning for CGM data analysis?
+Absolutely! Machine learning techniques can be powerful tools for predicting glucose levels and identifying patterns. Explore libraries like scikit-learn
and tensorflow
for advanced analysis.
How do I handle missing data in CGM datasets?
+Missing data can be imputed using various techniques, such as mean imputation or more advanced methods like KNN imputation. Choose an appropriate method based on the nature of your data and the impact of missing values on your analysis.